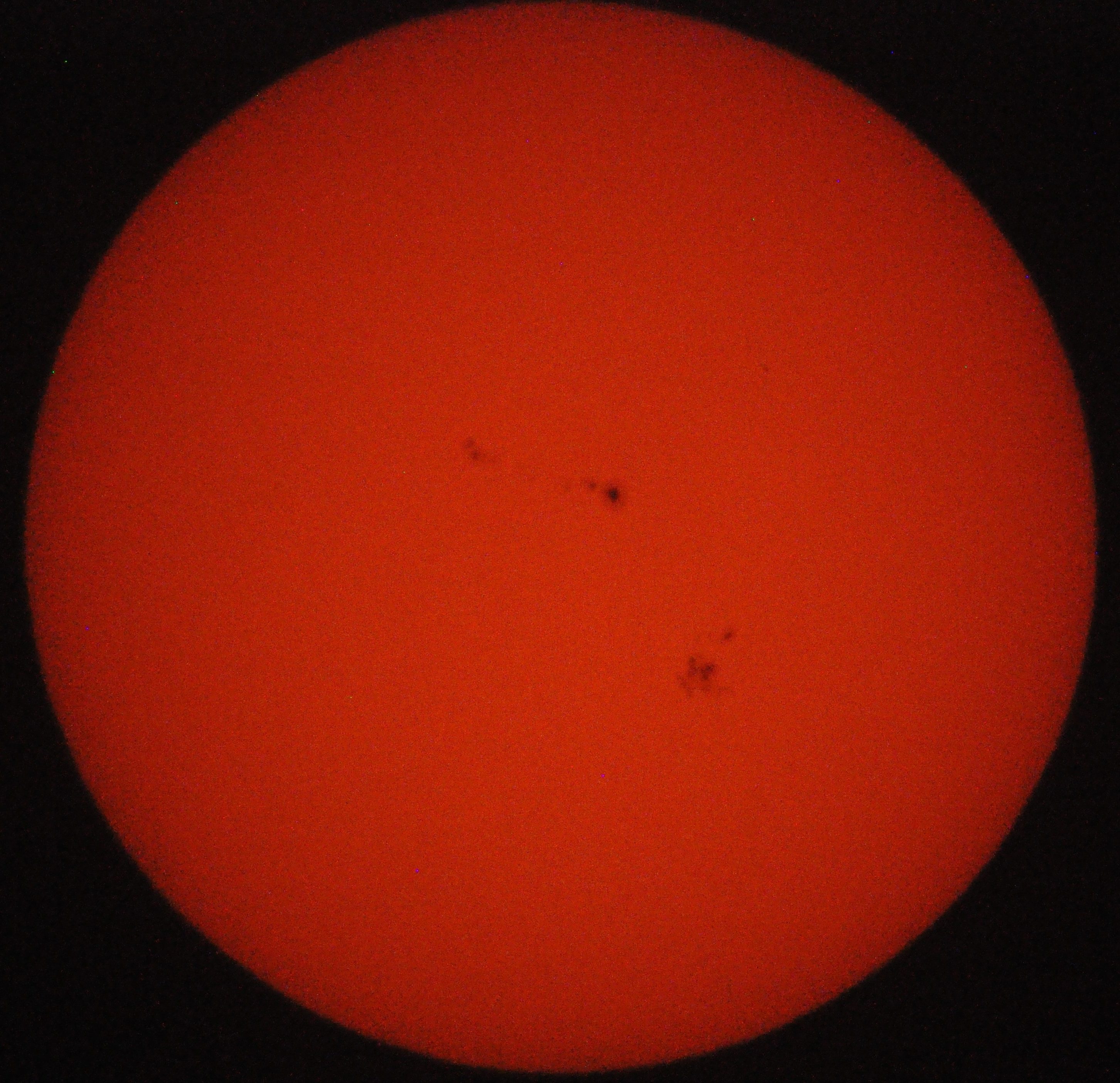
Here’s a photo I took of the sun using a homemade solar filter, showing sunspots 2673 (long streak just above the center) and 2674 (below and to the right of 2673). Compare with this photo from spaceweather.com.
The stuff in my head
Here’s a photo I took of the sun using a homemade solar filter, showing sunspots 2673 (long streak just above the center) and 2674 (below and to the right of 2673). Compare with this photo from spaceweather.com.
This is my first attempt at star trail photography. The photo was produced by stacking 48 one-minute exposures. It was a bit out of focus, I accidentally set the white balance on automatic, and I managed to bump the tripod partway through, but I still think it turned out well for a first attempt.
I also made a short time-lapse video from the photos used to create the star trail composite above. Shorter exposures (and more of them) would have made it smoother, but I still enjoy watching it.
I’m glad I decided to work from home today, and that the clouds cleared for a while. It meant that I got to view the partial solar eclipse, and I even managed to take a few pictures!
The picture above is a bit blurry, but you can clearly see the moon’s shadow, and even sunspots 2192 (the big cluster in the middle), 2193 (the tiny dark spot on the right), and 2194 (the tiny dark spot on the left). The sunspots aren’t remotely as clear as those same spots in this image on Spaceweather.com, but you can see their likeness.
The photo was taken with a Nikon D5000 DSLR, a Nikon 200mm lens, and a cheap welding helmet as the filter. I took several pictures but this was the least blurry. That lens is really difficult to focus sharply on something 93 million miles away.